Servo motors
Lesson #7—Giving your Arduino movementTheory
A servo motor is a motor with a built in control system (Fig. 1). It monitors its position and attempts to drive the axle to the commanded angle. Typical servo motors have approximately 180 degrees of rotational motion. Your Arduino can instruct the servo motor to move to (and hold) a particular angle.
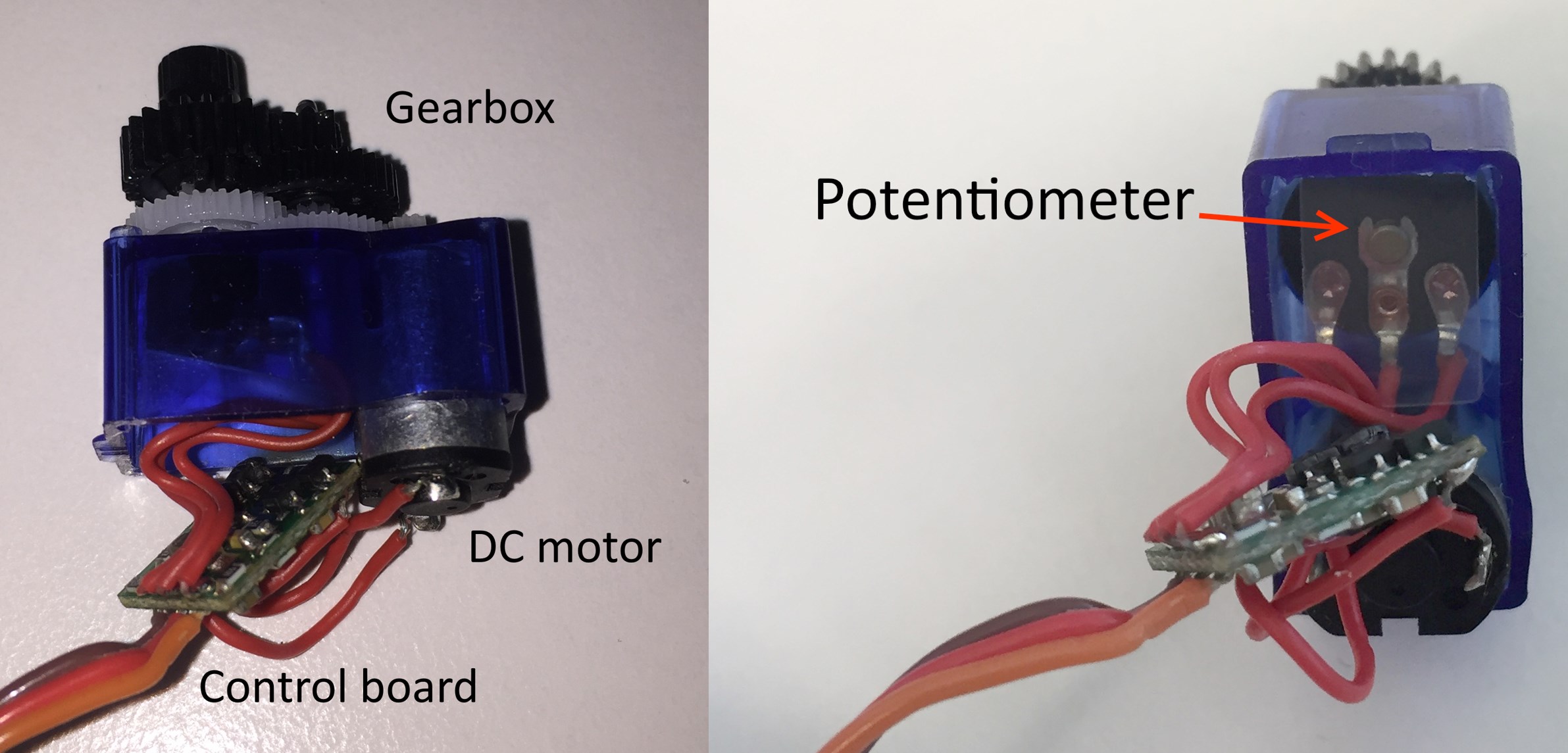
Fig. 1: Inside a small servo motor.
A servo motor has three wires attached:
- A 5 V supply,
- A ground, and
- A signal line that must run to a pin that is capable of outputting pulse width modulation (PWM). PWM-capable pins are labelled with a tilde (
~
).
The two most common colour schemes for these wires are shown below.
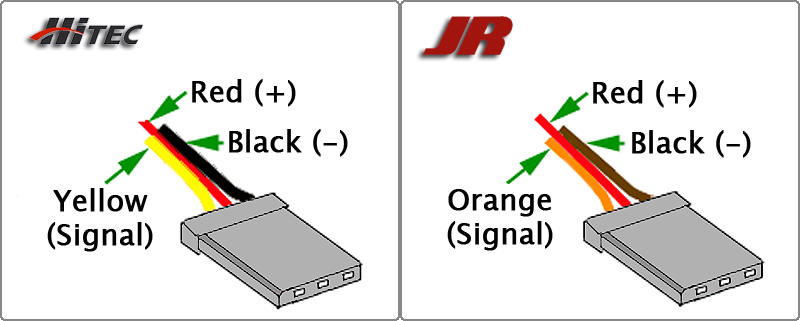
Fig. 2: Servo wire colour codes. Images by Randy Carr.
Wiring
Fig. 1: Schematic layout for the servo circuit.
Fig. 2: Breadboard layout for the servo circuit.
Code
#include <Servo.h>
Servo servo1;
void setup() {
// put your setup code here, to run once:
// Which pin is connected to the servo data line?
servo1.attach(5);
}
void loop() {
// put your main code here, to run repeatedly:
int angle;
// Set the angle of the servo
angle = 45; // degrees
// Send the command to the servo
servo1.write(angle);
// Wait a bit
delay(1000);
// Set a new angle
angle = 135; // degrees
// Send the command to the servo
servo1.write(angle);
// Wait a bit
delay(1000);
}
Exercise
Modify the above program so that the servo position is proportional to the analog signal measured from the LDR.
Imagine that your servo is connected to an instrument dial (like a car speedometer). Light intensity will be indicated by rotating the “needle.”
Topics for workshop discussion
- You can use a linear equation to relate analog value to rotational angle, e.g. \(y=mx+c\) where you choose appropriate values of \(m\) and \(c\).
- Servo motors are useful in small robotics applications, e.g. to move joints. There are many examples of 3D-printable servo-driven machines available online.
Technical aside on this circuit
This circuit powers the servo motor from the 5 V line on the Arduino. If you have a USB cable connected to your Arduino, the 5 V line is derived from the USB controller in the computer, and will supply up to 500 mA (assuming a USB 2.0 socket). If you have multiple motors or a larger servo, this might not be appropriate and you would need a dedicated power supply.